Configure mail service:
Go to the Felix Console - http://localhost:4502/system/console/configMgr
Search for Day CQ Mail Service
Enter the email server details as shown below and save the data (Here I am using Gmail server details).
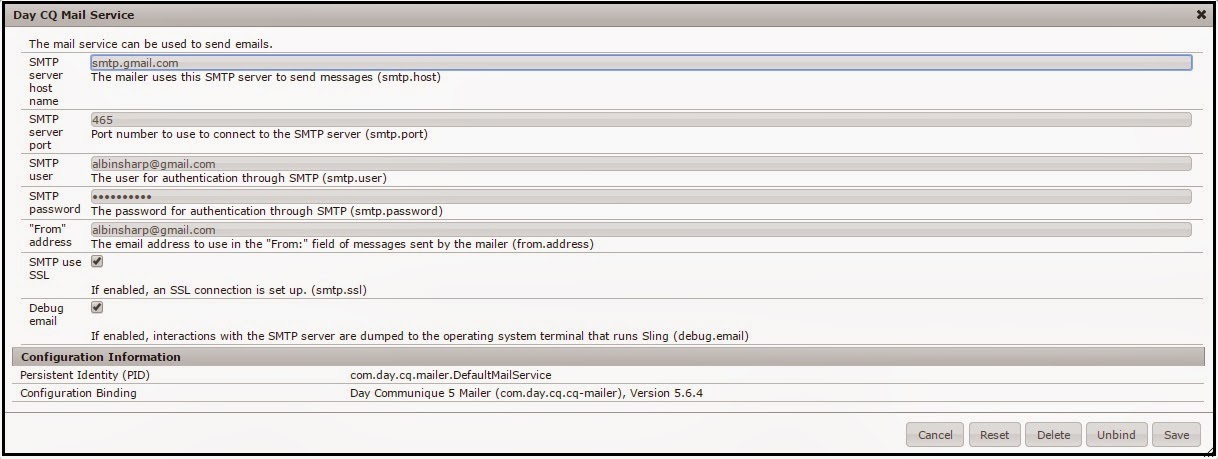
Email Template:
Create the email template as html file and store it in repository - /etc/email/template/emailTemplate.html (change the path accordingly)
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<body>
Hi ${firstName} ${lastName}</br>
This is the sample mail.
<ul>
#foreach( $data in $dataList )
<li>$data</li>
#end
</ul>
</body>
</html>
Maven dependencies to the POM.xml:
<dependency>
<groupId>com.day.cq</groupId>
<artifactId>cq-mailer</artifactId>
<version>5.4.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity</artifactId>
<version>1.6.2</version>
</dependency>
Import-Package configuration in POM.xml bundle plugin:
*,
org.apache.xerces.dom;resolution:=optional,org.apache.xerces.parsers;resolution:=optional,
oracle.xml.parser;resolution:=optional, oracle.xml.parser.v2;resolution:=optional,
org.jaxen;resolution:=optional, org.jaxen.jdom;resolution:=optional,
org.apache.xml.resolver;resolution:=optional,org.apache.xml.resolver.helpers;resolution:=optional,
org.apache.xml.resolver.tools;resolution:=optional,org.apache.tools.ant.launch;resolution:=optional,
org.apache.tools.ant.taskdefs.optional;resolution:=optional,org.apache.tools.ant.util.optional;resolution:=optional,
org.apache.avalon.framework.logger;resolution:=optional,sun.misc;resolution:=optional,
sun.rmi.rmic;resolution:=optional,sun.tools.javac;resolution:=optional,org.apache.bsf;resolution:=optional,
org.apache.env;resolution:=optional,org.apache.bcel.classfile;resolution:=optional,kaffe.rmi.rmic;resolution:=optional,
com.sun.jdmk.comm;resolution:=optional,com.sun.tools.javac;resolution:=optional,javax.jms;resolution:=optional,
antlr;resolution:=optional,antlr.collections.impl;resolution:=optional,org.jdom;resolution:=optional,
org.jdom.input;resolution:=optional,org.jdom.output;resolution:=optional,com.werken.xpath;resolution:=optional,
org.apache.tools.ant;resolution:=optional,org.apache.tools.ant.taskdefs;resolution:=optional,
org.apache.log;resolution:=optional,org.apache.log.format;resolution:=optional,org.apache.log.output.io;resolution:=optional,
<plugin>
<groupId>org.apache.felix</groupId>
<artifactId>maven-bundle-plugin</artifactId>
<extensions>true</extensions>
<configuration>
<instructions>
<Bundle-Activator>com.tr.commerce.connector.activator.Activator
</Bundle-Activator>
<Import-Package>
*,
org.apache.xerces.dom;resolution:=optional,org.apache.xerces.parsers;resolution:=optional,
oracle.xml.parser;resolution:=optional, oracle.xml.parser.v2;resolution:=optional,
org.jaxen;resolution:=optional, org.jaxen.jdom;resolution:=optional,
org.apache.xml.resolver;resolution:=optional,org.apache.xml.resolver.helpers;resolution:=optional,
org.apache.xml.resolver.tools;resolution:=optional,org.apache.tools.ant.launch;resolution:=optional,
org.apache.tools.ant.taskdefs.optional;resolution:=optional,org.apache.tools.ant.util.optional;resolution:=optional,
org.apache.avalon.framework.logger;resolution:=optional,sun.misc;resolution:=optional,
sun.rmi.rmic;resolution:=optional,sun.tools.javac;resolution:=optional,org.apache.bsf;resolution:=optional,
org.apache.env;resolution:=optional,org.apache.bcel.classfile;resolution:=optional,kaffe.rmi.rmic;resolution:=optional,
com.sun.jdmk.comm;resolution:=optional,com.sun.tools.javac;resolution:=optional,javax.jms;resolution:=optional,
antlr;resolution:=optional,antlr.collections.impl;resolution:=optional,org.jdom;resolution:=optional,
org.jdom.input;resolution:=optional,org.jdom.output;resolution:=optional,com.werken.xpath;resolution:=optional,
org.apache.tools.ant;resolution:=optional,org.apache.tools.ant.taskdefs;resolution:=optional,
org.apache.log;resolution:=optional,org.apache.log.format;resolution:=optional,org.apache.log.output.io;resolution:=optional,
</Import-Package>
<Bundle-SymbolicName>email-template
</Bundle-SymbolicName>
<Bundle-Vendor>Albin</Bundle-Vendor>
<Bundle-Category>email</Bundle-Category>
<Embed-Directory>dependencies</Embed-Directory>
<Embed-Transitive>true</Embed-Transitive>
</instructions>
</configuration>
</plugin>
Make sure the below plugin is configured in pom.xml(to add the dependencies to bundle classpath)
<plugin>
<groupId>org.apache.felix</groupId>
<artifactId>maven-bundle-plugin</artifactId>
<version>2.3.7</version>
<configuration>
<instructions>
<Embed-Dependency>*;scope=compile|runtime</Embed-Dependency>
<Embed-Directory>OSGI-INF/lib</Embed-Directory>
<Embed-Transitive>true</Embed-Transitive>
</instructions>
</configuration>
</plugin>
Create the Email servlet:
Create a servlet to send the email with the provides details.
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.StringWriter;
import java.util.ArrayList;
import javax.jcr.Node;
import javax.jcr.Session;
import javax.mail.internet.InternetAddress;
import javax.servlet.Servlet;
import javax.servlet.ServletException;
import org.apache.commons.mail.HtmlEmail;
import org.apache.felix.scr.annotations.Component;
import org.apache.felix.scr.annotations.Reference;
import org.apache.felix.scr.annotations.Service;
import org.apache.felix.scr.annotations.sling.SlingServlet;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.SlingHttpServletResponse;
import org.apache.sling.api.servlets.SlingAllMethodsServlet;
import org.apache.sling.jcr.api.SlingRepository;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.Velocity;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.day.cq.mailer.MessageGateway;
import com.day.cq.mailer.MessageGatewayService;
@SuppressWarnings({ "serial" })
@Component(metatype = false)
@SlingServlet(name = "EmailServlet", description = "EmailServlet", methods = "GET", generateComponent =false, paths = "/services/EmailServlet")
@Service(Servlet.class)
public class EmailService extends SlingAllMethodsServlet
{
private static final Logger LOG = LoggerFactory.getLogger(EmailService.class);
@Reference
private SlingRepository repository;
@Reference
private MessageGatewayService messageGatewayService;
@Override
protected void doGet(SlingHttpServletRequest request,
SlingHttpServletResponse response) throws ServletException,
IOException {
String results = sendMail("albinsharp@gmail.com", "albinsharp@gmail.com");
if (results != null && results.equalsIgnoreCase("success")) {
response.getWriter().write("Email Send Successfully");
} else {
response.getWriter().write(results);
}
}
public String sendMail(String fromAddress, String toEmailAddress) {
ArrayList<InternetAddress> emailRecipients = new ArrayList<InternetAddress>();
Session session = null;
String templateLink = "/etc/email/template/mailtemplate.html";
String result="success";
try {
session = repository.loginAdministrative(null);
String templateReference = templateLink.substring(1)+"/jcr:content";
Node root = session.getRootNode();
Node jcrContent = root.getNode(templateReference);
InputStream is = jcrContent.getProperty("jcr:data").getBinary().getStream();
InputStreamReader reader = new InputStreamReader(is);
VelocityContext context = new VelocityContext();
context.put("firstName", "Albin");
context.put("lastName", "Issac");
ArrayList<String> dataList=new ArrayList<String>();
dataList.add("Test1");
dataList.add("Test2");
dataList.add("Test3");
context.put("dataList", dataList);
StringWriter swOut = new StringWriter();
Velocity.evaluate(context, swOut, "LOG", reader);
LOG.info("Email content.."+swOut.toString());
HtmlEmail email = new HtmlEmail();
emailRecipients.add(new InternetAddress(toEmailAddress));
email.setCharset("UTF-8");
email.setFrom(fromAddress);
email.setTo(emailRecipients);
email.setSubject("This is the test mail");
email.setHtmlMsg(swOut.toString());
MessageGateway<HtmlEmail> messageGateway = this.messageGatewayService.getGateway(HtmlEmail.class);
messageGateway.send(email);
emailRecipients.clear();
} catch (Exception e) {
result="Error in sending Email.."+e.getMessage();
}finally
{
session.logout();
}
return result;
}
}
No comments:
Post a Comment
If you have any doubts or questions, please let us know.