Models
While most of what I have written so far has been focused on the code itself, workflows are very hard to code manually so my recommendation is to log in to the editor and create/modify your workflows in the editor. As it is visual it is much easier to use, and the resulting code can be exported from the package manager and added to your content package
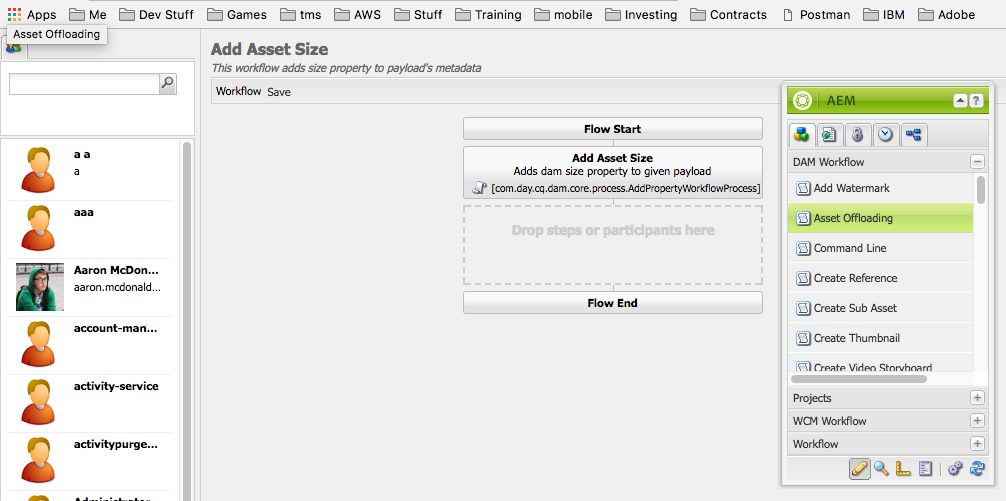
Workflow Edit
While most of what I have written so far has been focused on the code itself, workflows are very hard to code manually so my recommendation is to log in to the editor and create/modify your workflows in the editor. As it is visual it is much easier to use, and the resulting code can be exported from the package manager and added to your content package
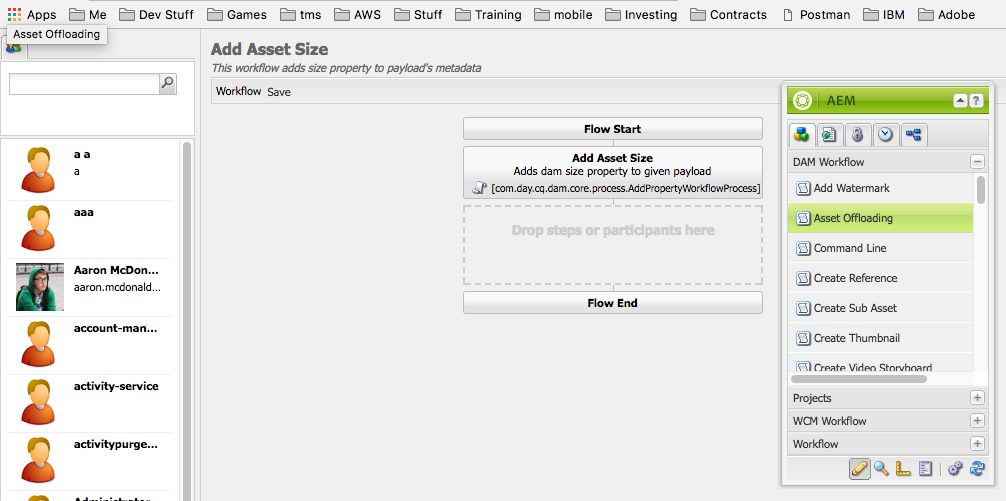
Workflow Edit
AEM comes with a set of custom made components for the workflow, and while it is possible to create your own, that is beyond the scope of this discussion.
The default workflow steps that come with AEM are:
The default workflow steps that come with AEM are:
- DAM Workflow
- Steps for creating/modifying images in the dam
- Projects
- Steps for adding photo processing
- WCM Workflow
- Steps for activation/deactivation of pages both forward and reverse
- Workflow
- The main workflow steps
Workflow steps
The workflow steps are the main area of the workflow dialogs that i am going to discuss as these are the ones that are really of interest to most people.
The workflow steps are the main area of the workflow dialogs that i am going to discuss as these are the ones that are really of interest to most people.
AND Split
As you can guess, the AND Split allows you to split the workflow in more than one flow. This is used if you have multiple tasks that need to occur at the same time.
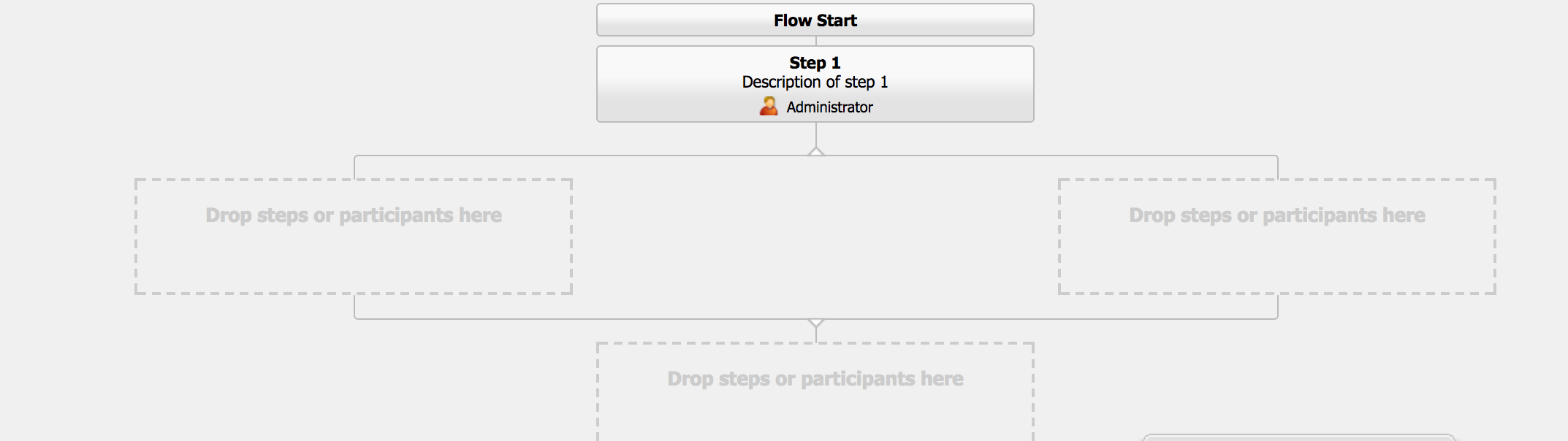
As you can guess, the AND Split allows you to split the workflow in more than one flow. This is used if you have multiple tasks that need to occur at the same time.
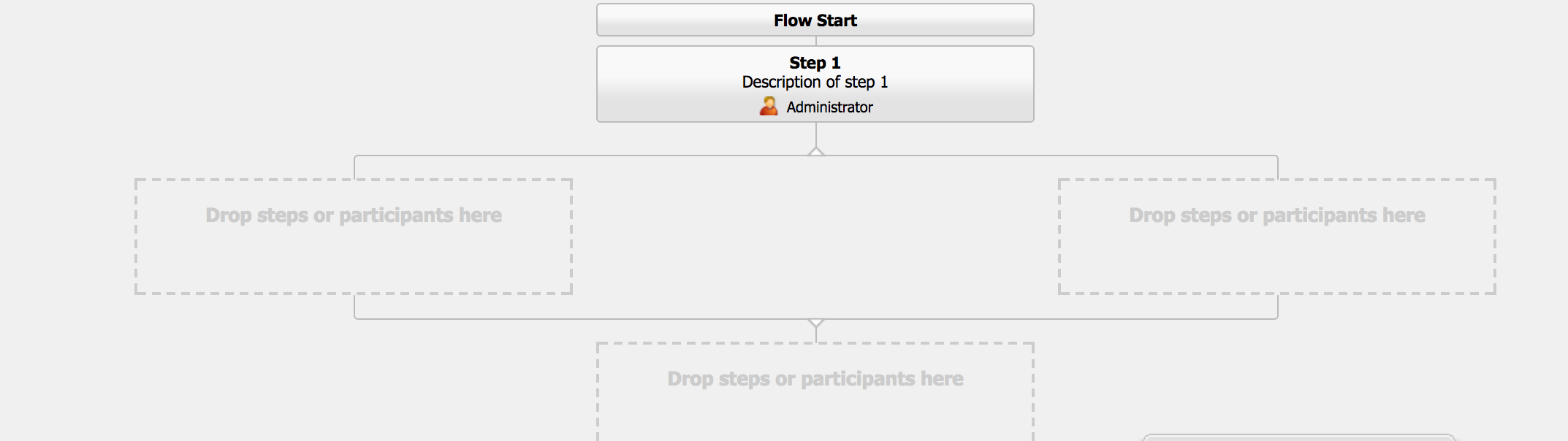
AND Workflow 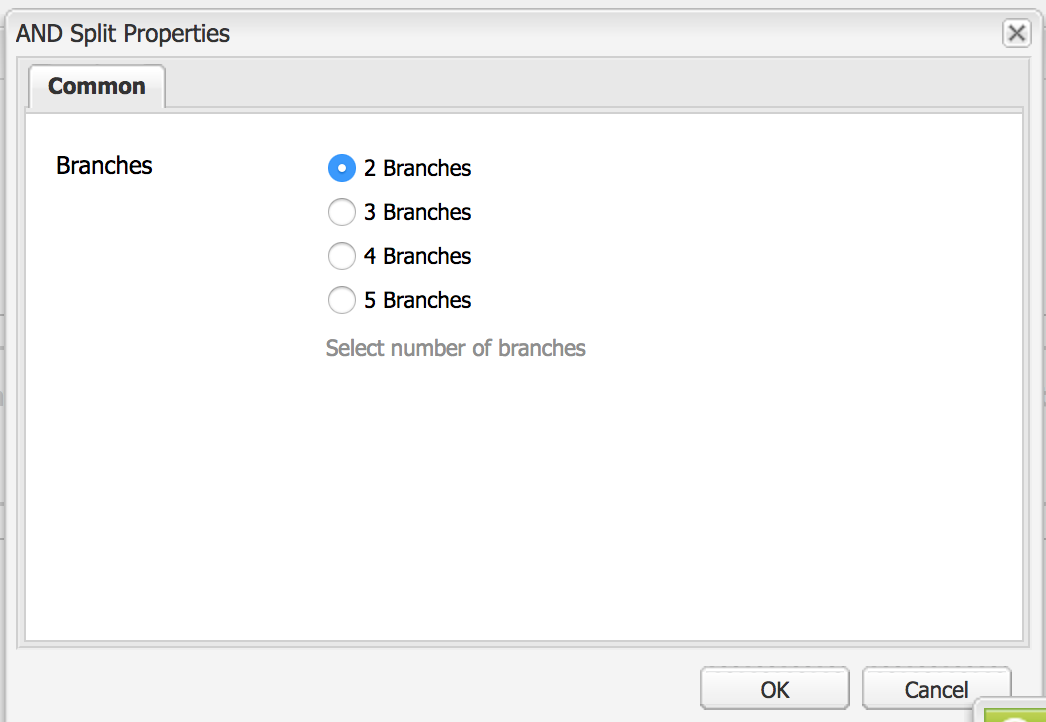
AND Dialog
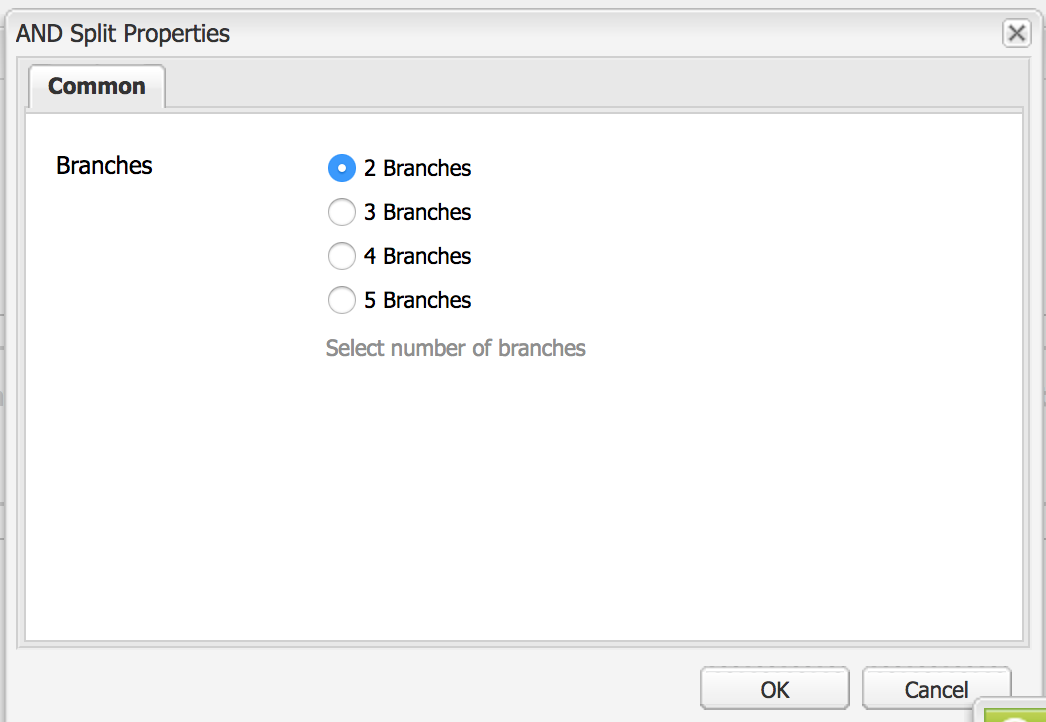
AND Dialog
By default, the AND flow splits into 2 branches, but you can have upto 5 branches running at the same time. The more branches, the more complex your flow will become especially when you have manual steps involved.
Absolute Timer Auto Advancer
There are no properties for the Absolute Timer Auto Advancer. It looks to see a property called "absoluteTime" in the workflow's metadata and it uses that as the time that it will advance to the next step
There are no properties for the Absolute Timer Auto Advancer. It looks to see a property called "absoluteTime" in the workflow's metadata and it uses that as the time that it will advance to the next step
Auto Advancer
There is little to no documentation on the auto advancer, to everything I can tell it is the base type for all other auto advancers
There is little to no documentation on the auto advancer, to everything I can tell it is the base type for all other auto advancers
Call Url
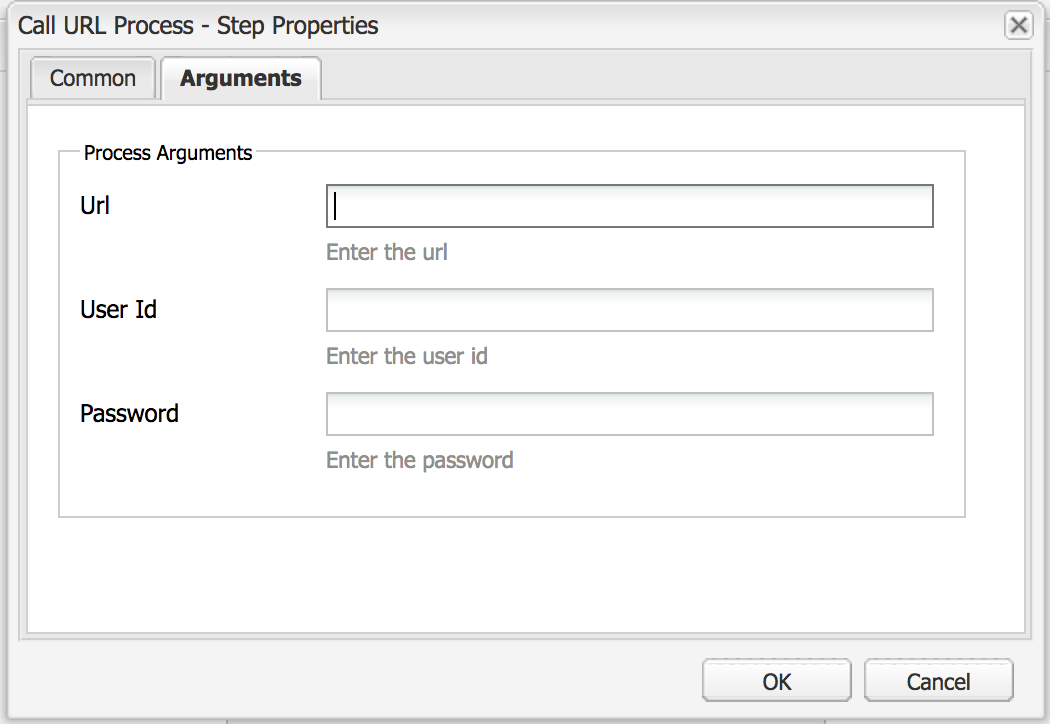
Call URL
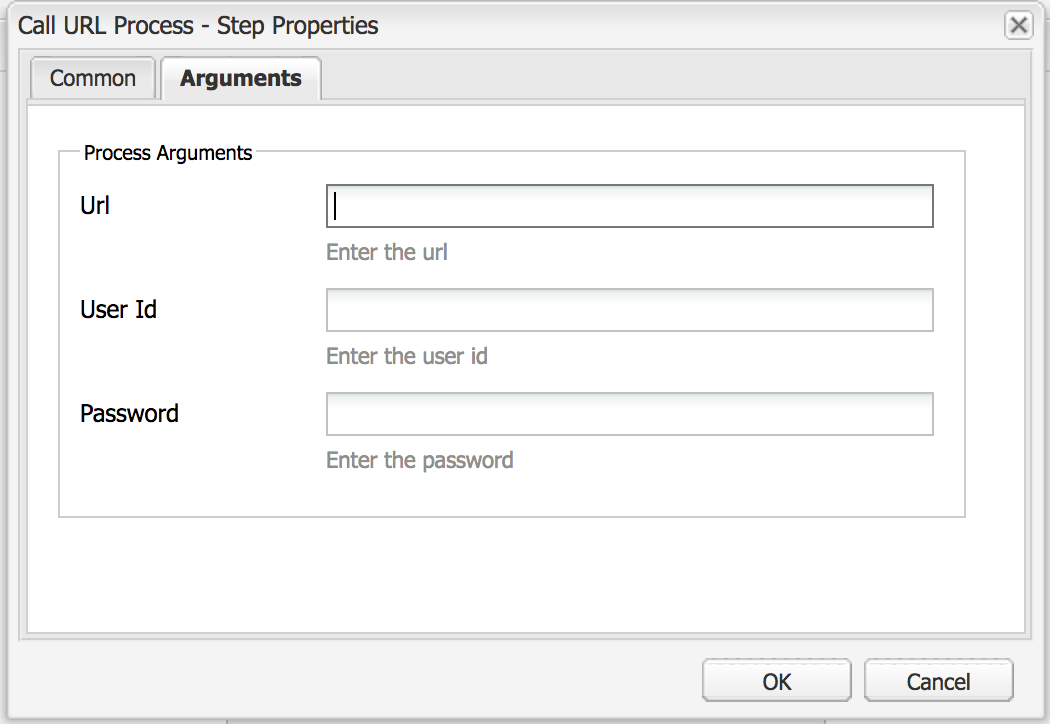
Call URL
As expected the Call Url step allows you to make an external callout from AEM
Container Step
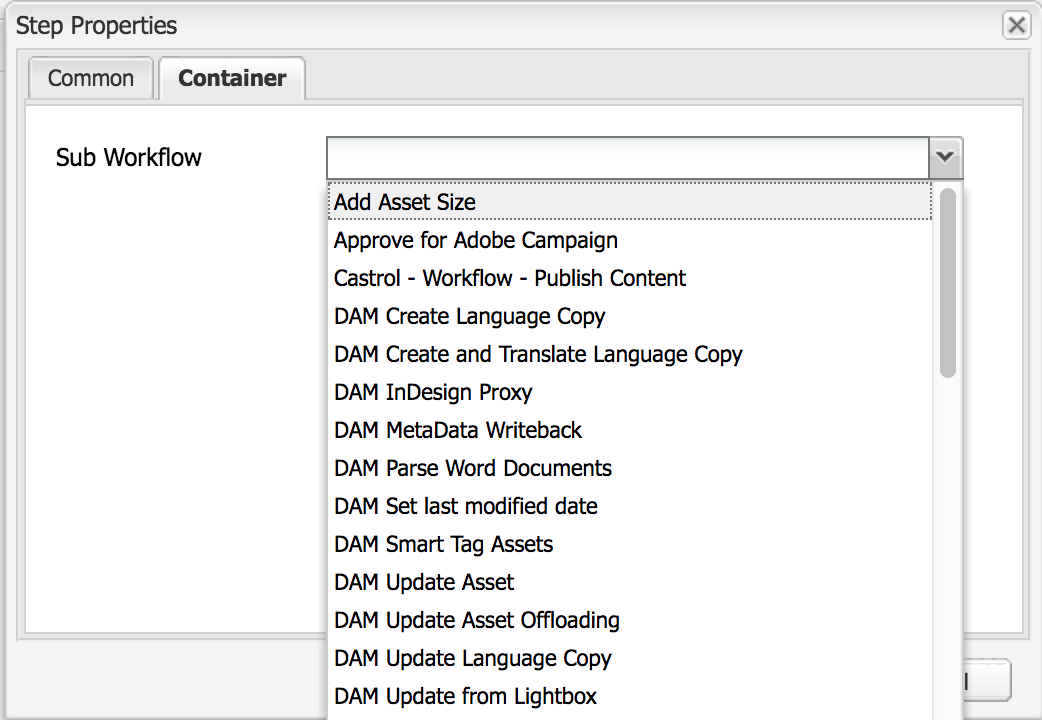
Container Step
Container Step
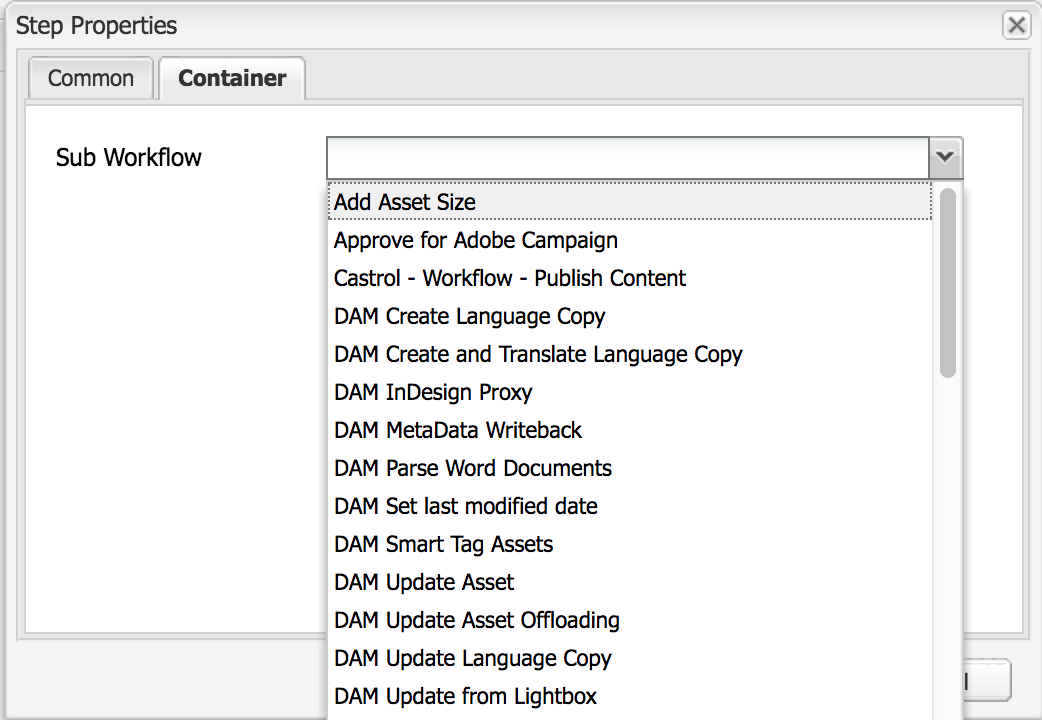
Container Step
The container step allows the workflow to run another workflow
Create Task
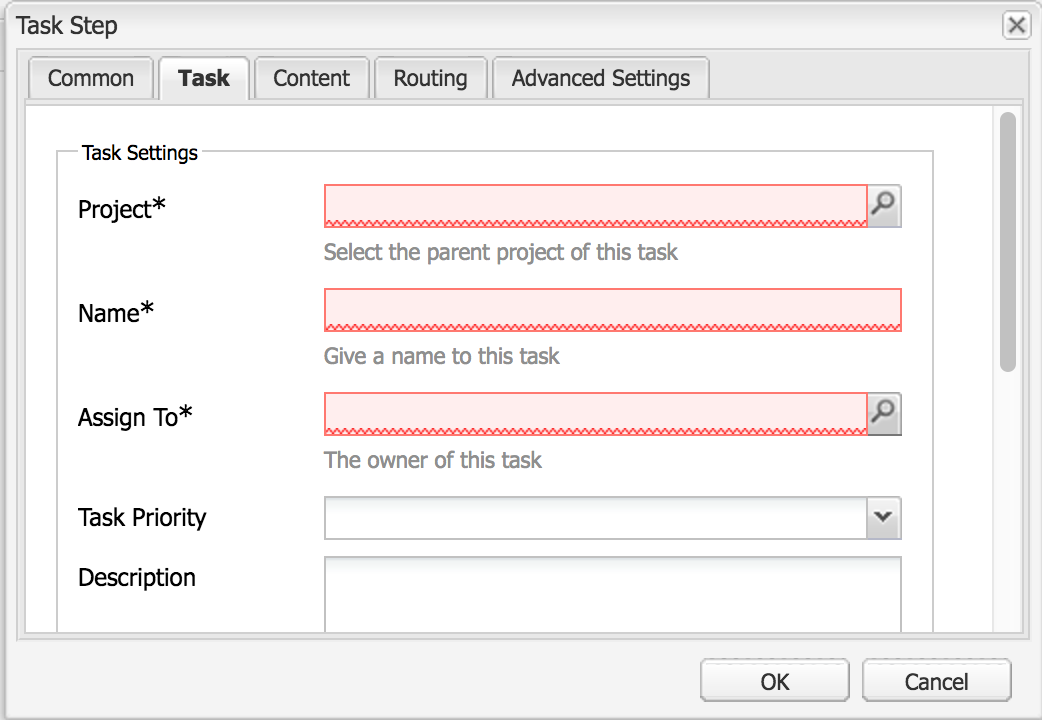
Create Task
Create Task
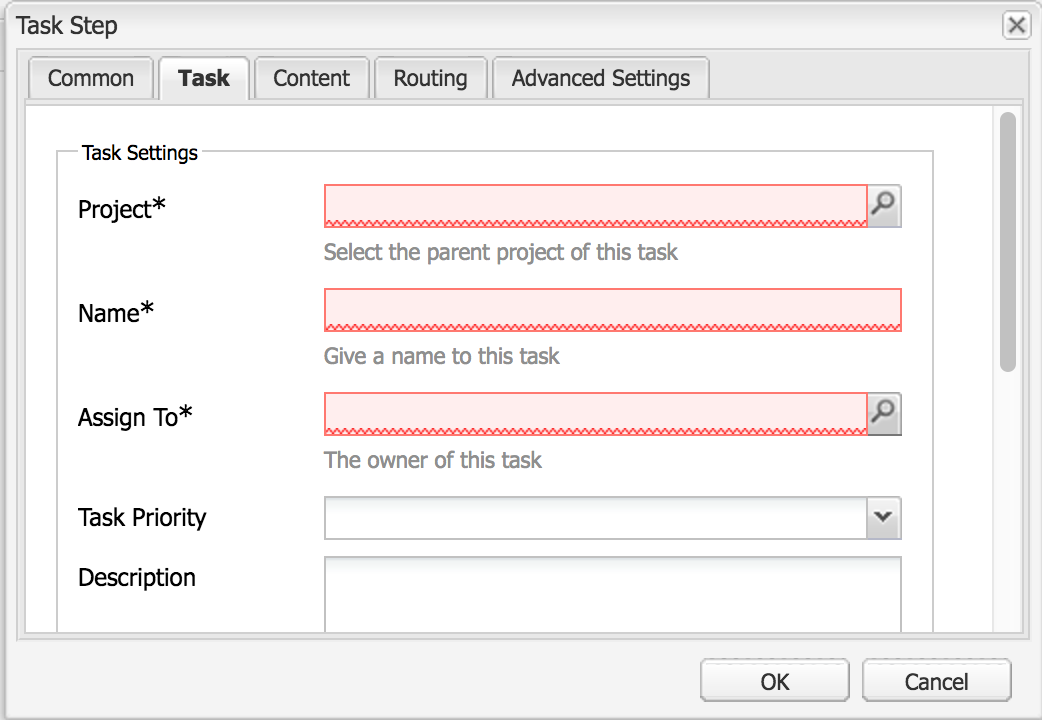
Create Task
The create task step creates a task in a specific user or group's inbox
Delete Node
The Delete node step will remove the workflow's data node. This can be used as a cleanup task
The Delete node step will remove the workflow's data node. This can be used as a cleanup task
Dialog Participant Step
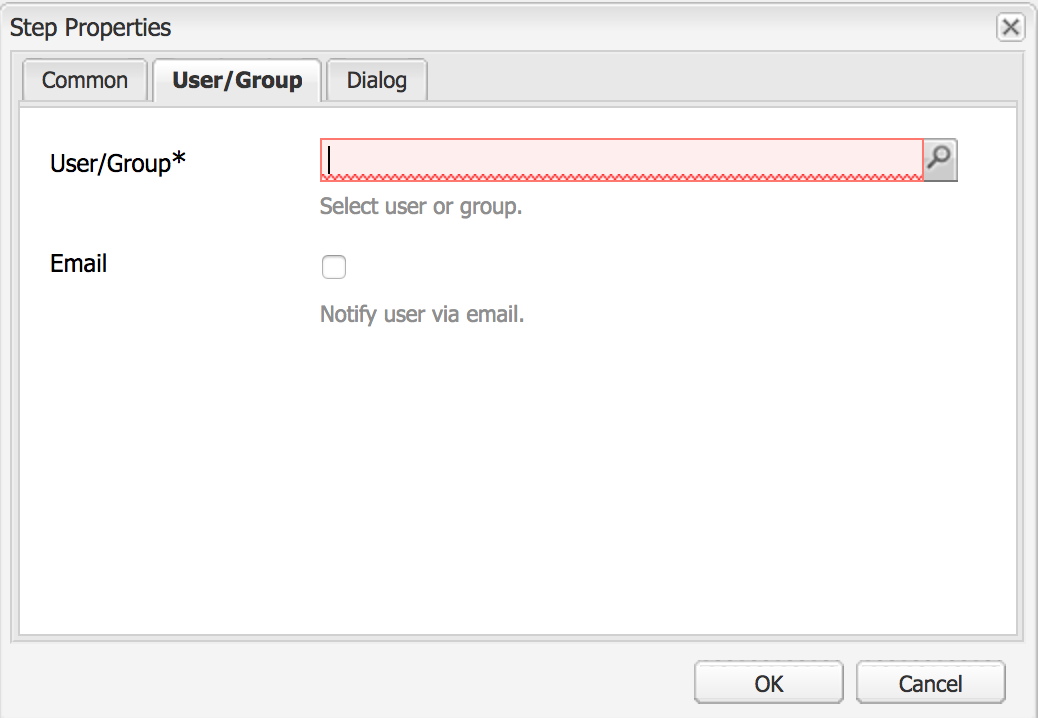
Participant Step
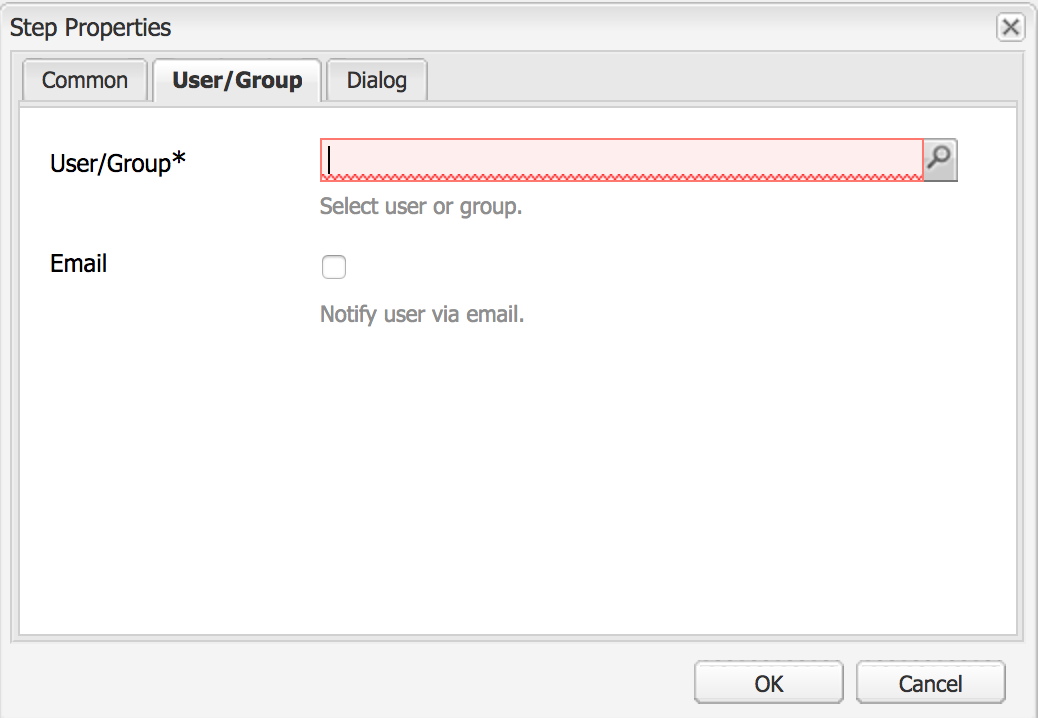
Participant Step
The Dialog Participant step allows you to choose a user/group for the next step, and a dialog for the user(s) to fill in
Dynamic Participant Step
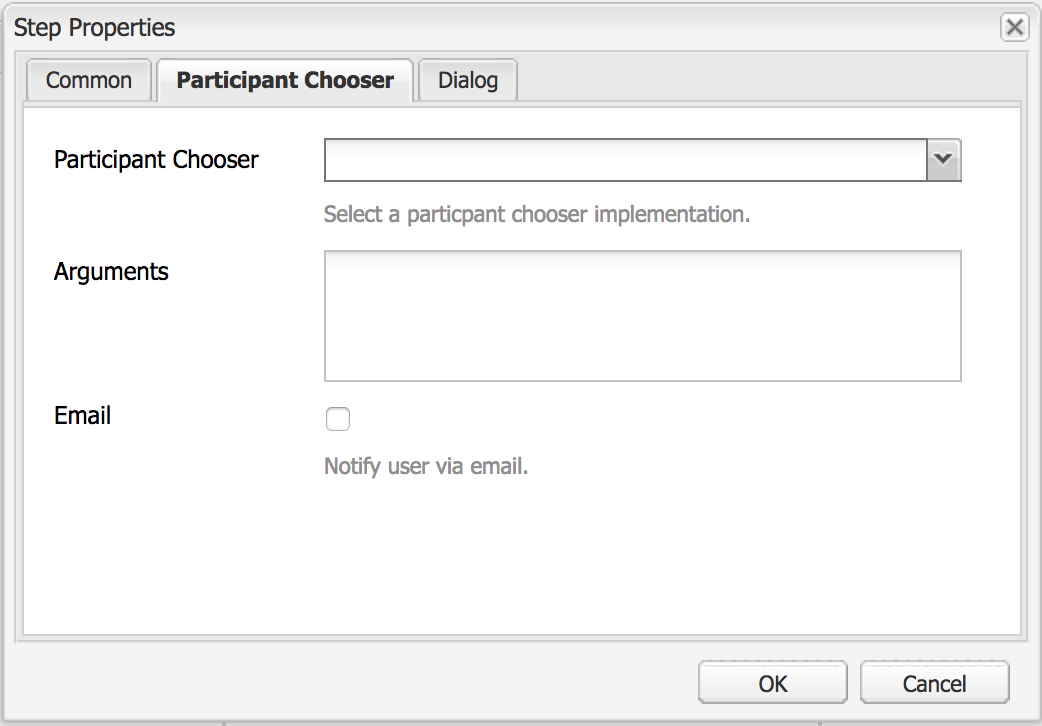
Dynamic Participant Step
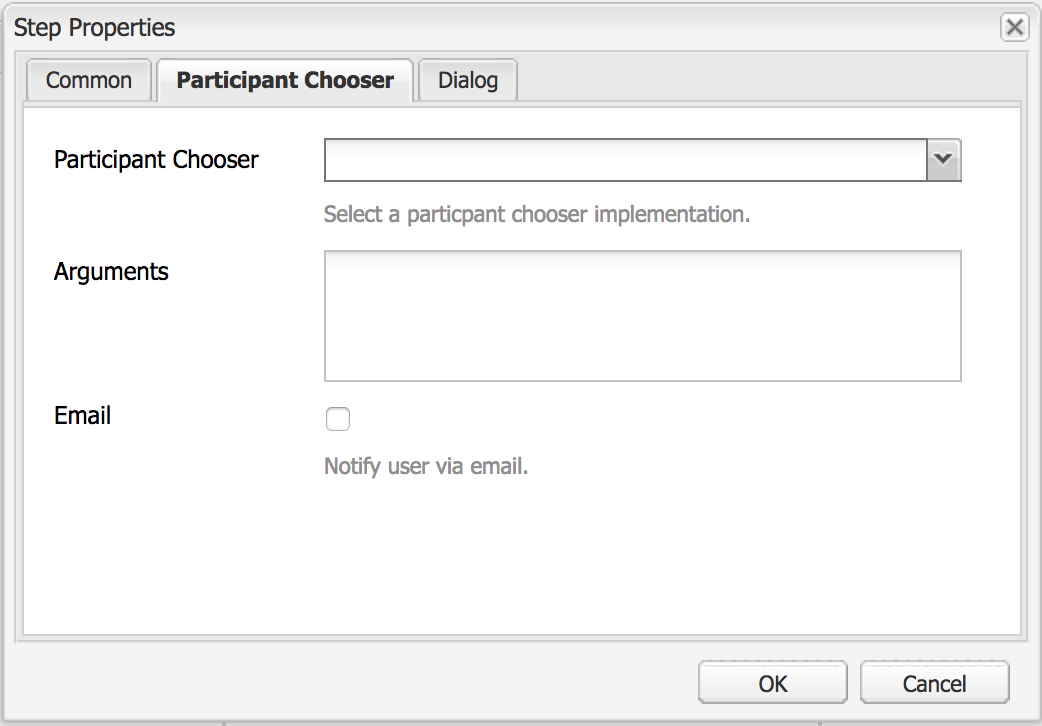
Dynamic Participant Step
Just like the Dialog participant step, this allows the user to enter a dialog (optional), however, the choice of user/group is done in code by a Participant chooser. There are some pre-configured choosers or you can write your own
package sample.core.services;
import org.apache.felix.scr.annotations.Component;
import org.apache.felix.scr.annotations.Properties;
import org.apache.felix.scr.annotations.Property;
import org.apache.felix.scr.annotations.Reference;
import org.apache.felix.scr.annotations.Service;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.api.resource.ResourceResolverFactory;
import org.osgi.framework.Constants;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.day.cq.workflow.WorkflowException;
import com.day.cq.workflow.WorkflowSession;
import com.day.cq.workflow.exec.ParticipantStepChooser;
import com.day.cq.workflow.exec.WorkItem;
import com.day.cq.workflow.metadata.MetaDataMap;
@Component (immediate = true )
@ServiceM
@Properties ({
@Property (name = Constants.SERVICE_DESCRIPTION, value = "Sample - Participant Chooser" ),
@Property (name = Constants.SERVICE_VENDOR, value = "Sample" )}) public class ParticipantStepChooserImpl implements ParticipantStepChooser {
private static Logger logger = LoggerFactory.getLogger(ParticipantStepChooserImpl. class );
private static int pos = 0 ;
@Override
public String getParticipant(WorkItem item, WorkflowSession session, MetaDataMap map) throws WorkflowException {
if (pos == 0 ) {
return "admin" } else if (pos == 1 ) {
return "sample-authors" ; }
pos++;
return "other-users" } }
package sample.core.services;
import org.apache.felix.scr.annotations.Component;
import org.apache.felix.scr.annotations.Properties;
import org.apache.felix.scr.annotations.Property;
import org.apache.felix.scr.annotations.Reference;
import org.apache.felix.scr.annotations.Service;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.api.resource.ResourceResolverFactory;
import org.osgi.framework.Constants;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.day.cq.workflow.WorkflowException;
import com.day.cq.workflow.WorkflowSession;
import com.day.cq.workflow.exec.ParticipantStepChooser;
import com.day.cq.workflow.exec.WorkItem;
import com.day.cq.workflow.metadata.MetaDataMap;
@Component (immediate = true )
@ServiceM
@Properties ({
@Property (name = Constants.SERVICE_DESCRIPTION, value = "Sample - Participant Chooser" ),
@Property (name = Constants.SERVICE_VENDOR, value = "Sample" )}) public class ParticipantStepChooserImpl implements ParticipantStepChooser {
private static Logger logger = LoggerFactory.getLogger(ParticipantStepChooserImpl. class );
private static int pos = 0 ;
@Override
public String getParticipant(WorkItem item, WorkflowSession session, MetaDataMap map) throws WorkflowException {
if (pos == 0 ) {
return "admin" } else if (pos == 1 ) {
return "sample-authors" ; }
pos++;
return "other-users" } }
As you can see programmatically you can tell the workflow which user or user group is required to activate the next step
Extract Export Data
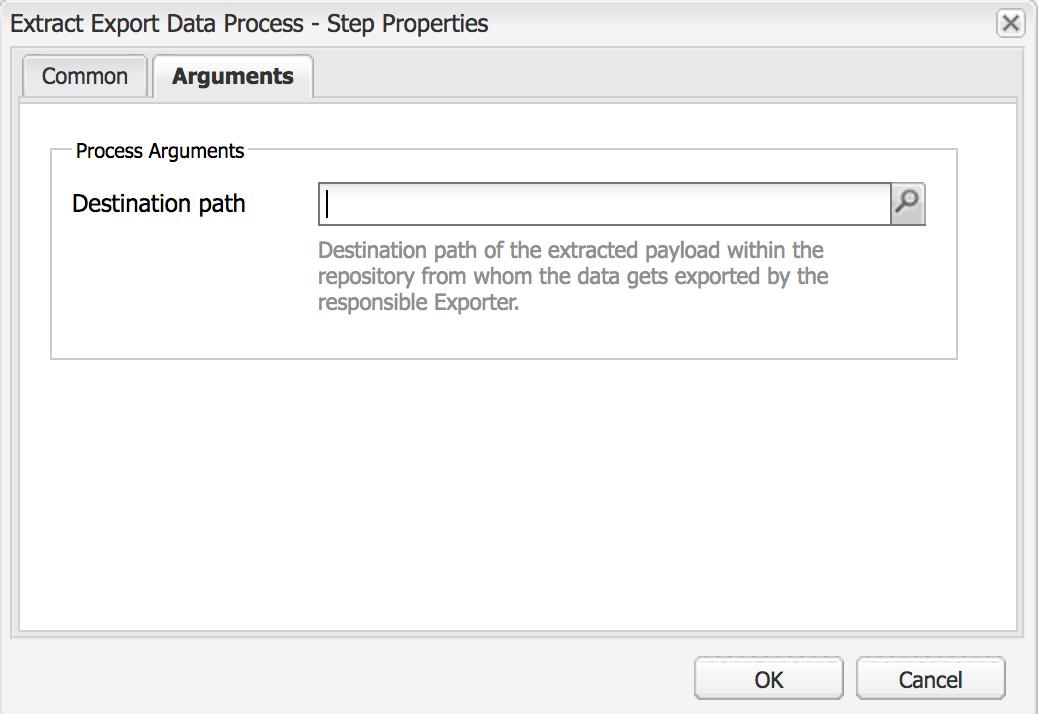
Extract Export Data
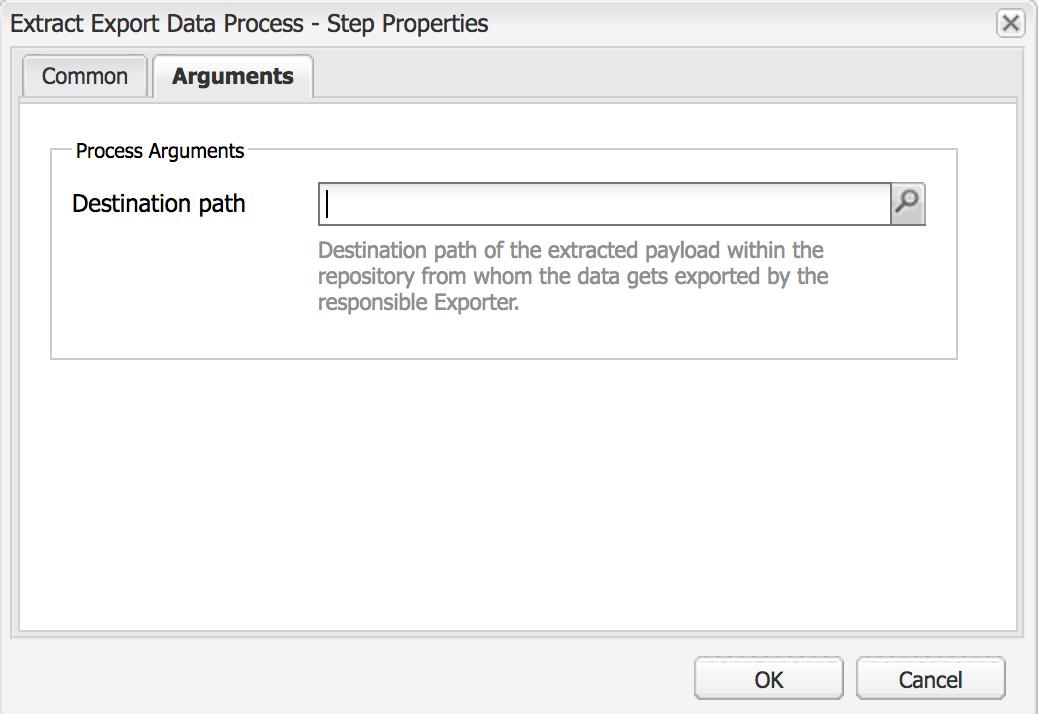
Extract Export Data
The extract export data step will take the data in the workflow and export it into the jcr:repository at the specified destination
Form Participant Step
This is very similar to the Dialog Participant Step, but instead of selecting a dialog you choose an AEM form for the user to fill in
This is very similar to the Dialog Participant Step, but instead of selecting a dialog you choose an AEM form for the user to fill in
Generic Job Process
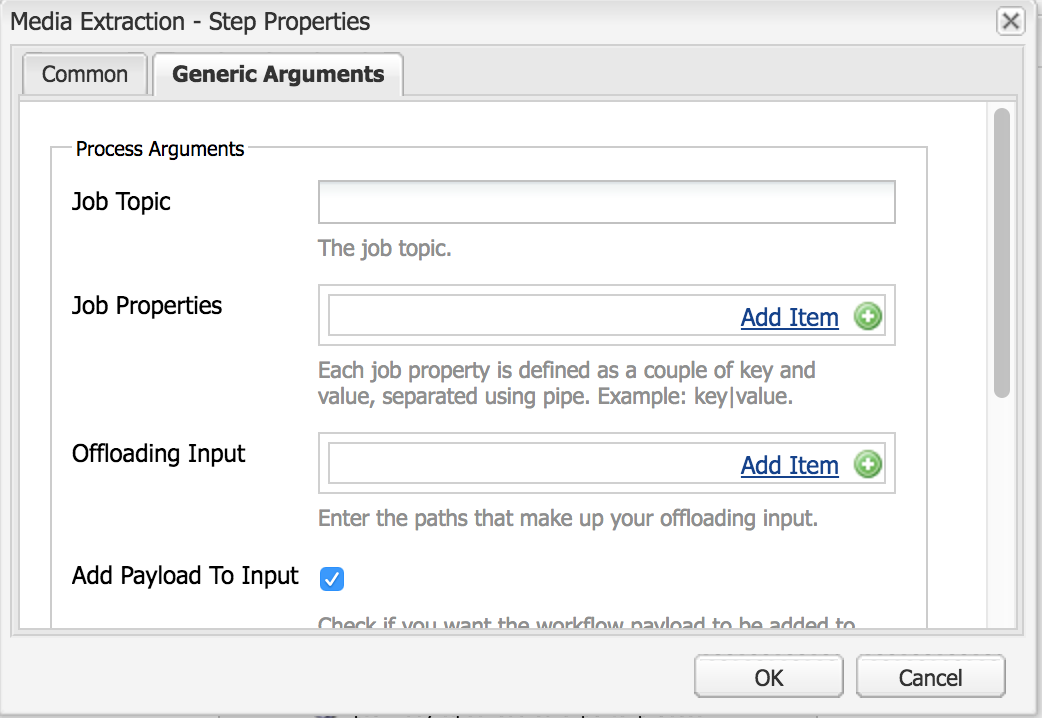
Generic Job Process
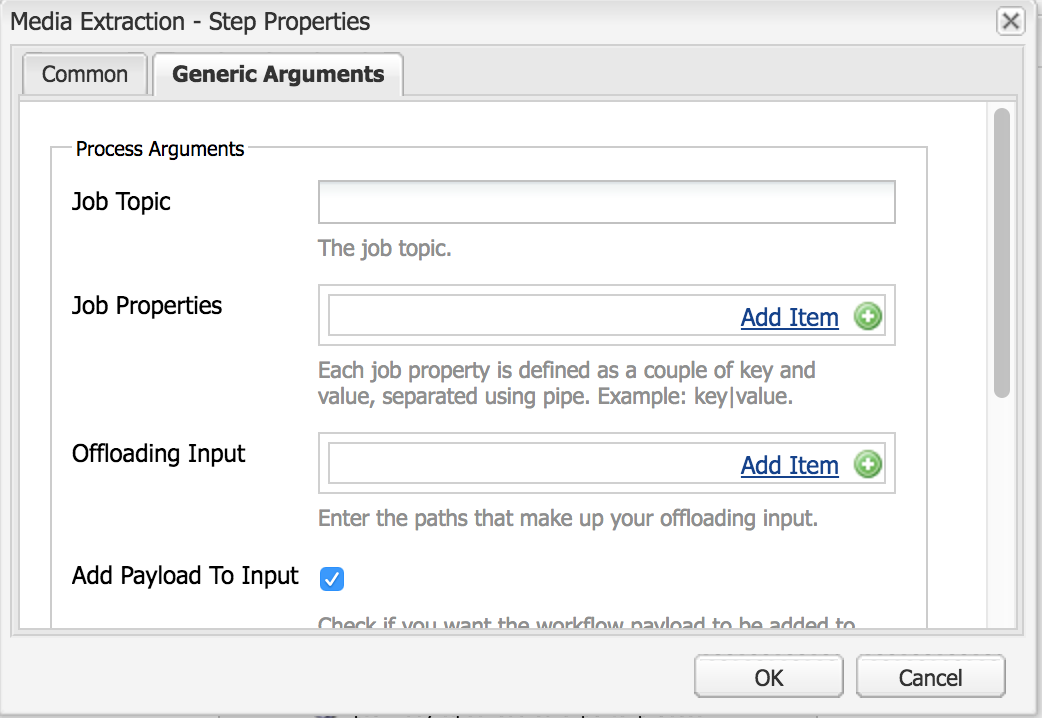
Generic Job Process
The Generic Job process allows the workflow to add a job into a queue. It will wait for the job process to complete and potentially update the workflow metadata but can continue on a timeout if required
Goto Step
Goto Step
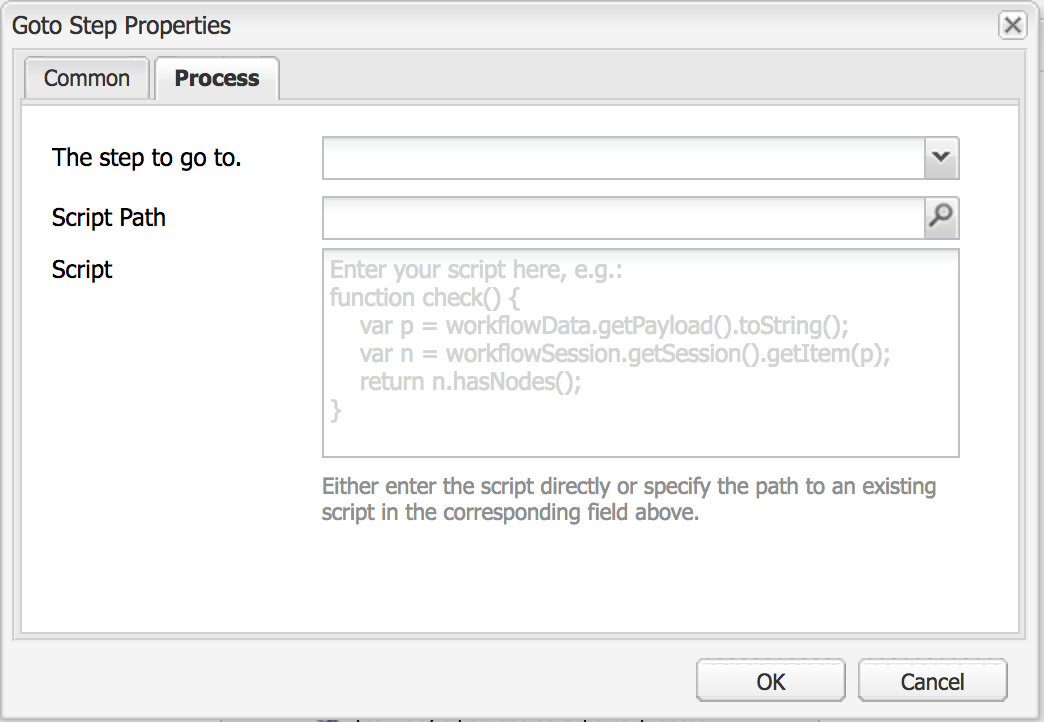
Then go to step allows you to either choose a step to go to in another part of the flow or to programmatically via the script to choose what the next step is
Lock Payload Process
The lock payload process will lock the workflow's payload meaning no other step can modify the payload until it is unlocked
The lock payload process will lock the workflow's payload meaning no other step can modify the payload until it is unlocked
No Operation
The no operation doesn't do anything, it is used mainly in the OR Split to make a fallout flow
The no operation doesn't do anything, it is used mainly in the OR Split to make a fallout flow
OR Split
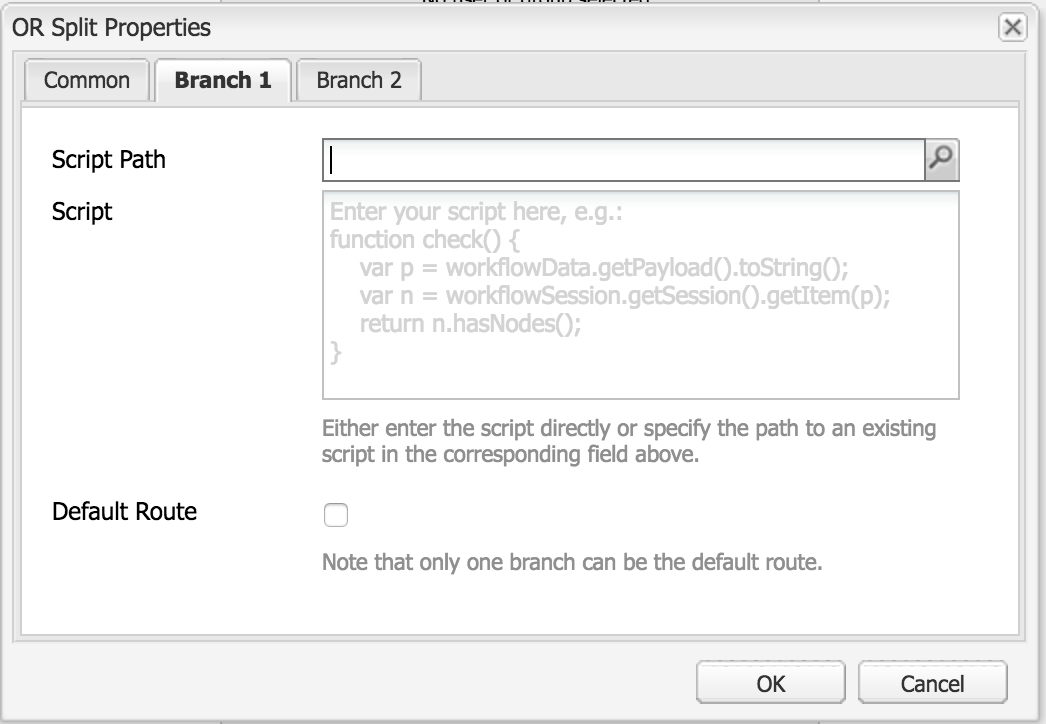
OR
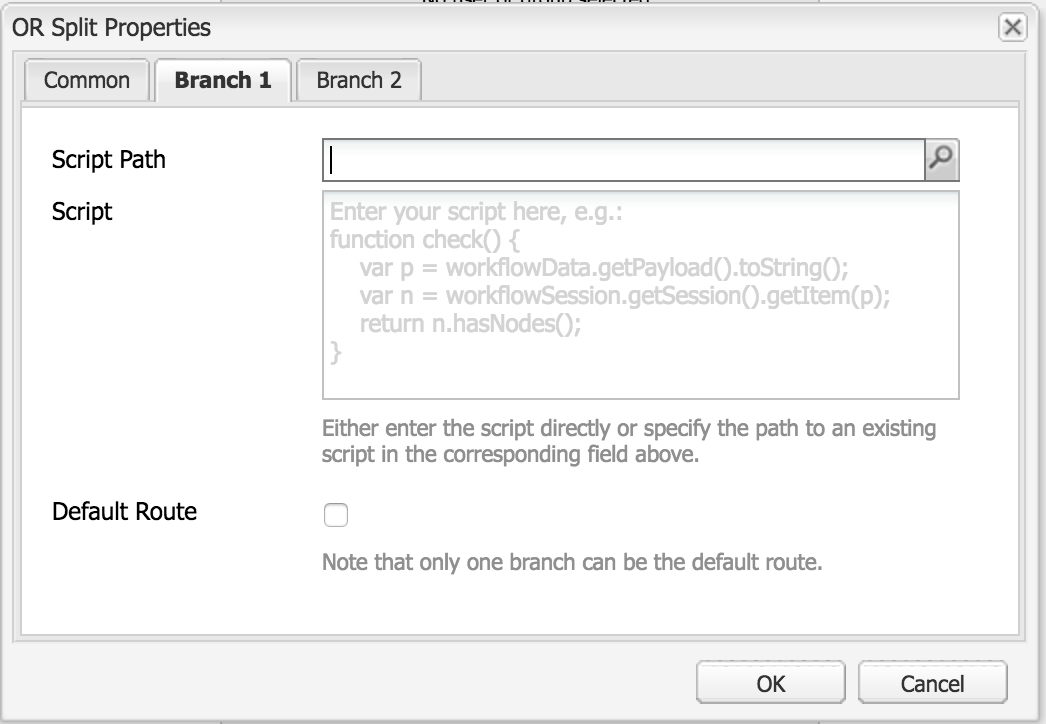
OR
Like the AND Split, the or split can have between 2 and 5 branches, which branch is taken is decided by the script on each branch, starting with branch 1 e.t.c Only a single branch can run. If no branch returns true then it will go to the default route if one is set, otherwise, the OR Split will error.
Participant Step
Like the other participant steps, this allows you to select the user or group that needs to interact with the workflow next
Like the other participant steps, this allows you to select the user or group that needs to interact with the workflow next
Random Participant Chooser
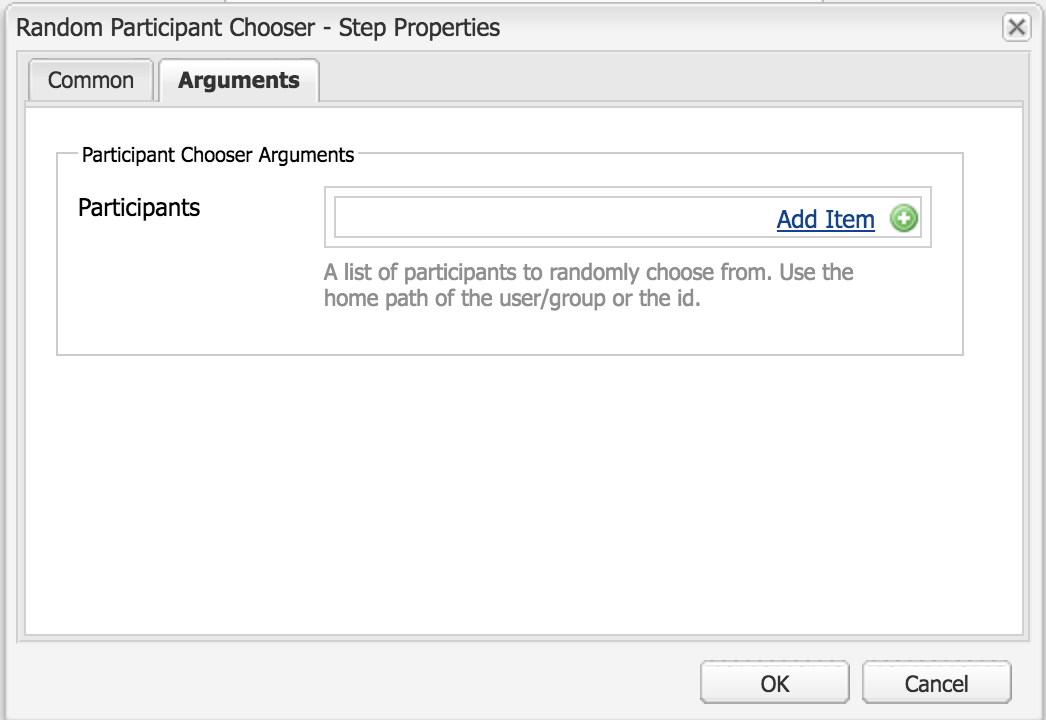
Random Participant Chooser
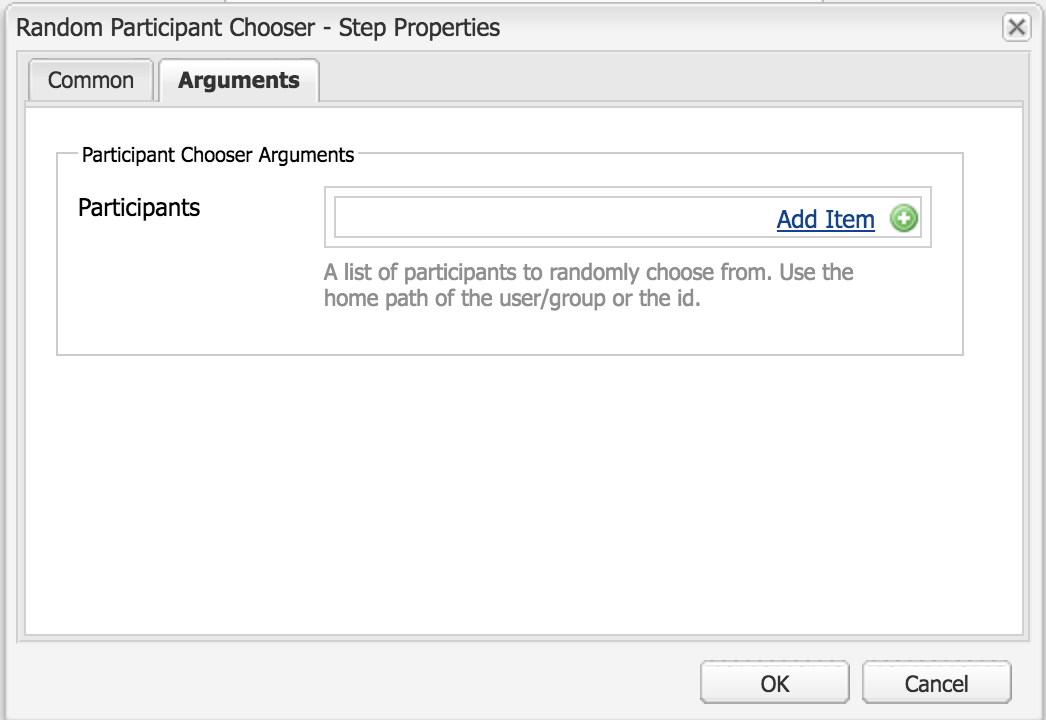
Random Participant Chooser
The Random Participant Chooser allows you to create a list of users, and it will randomly pick one of them to be the user that needs to interact with the workflow next
Scene7
There isn't any documentation on what this step does
Test & Target Offer
There isn't any documentation on what this step does
Test & Target Offer
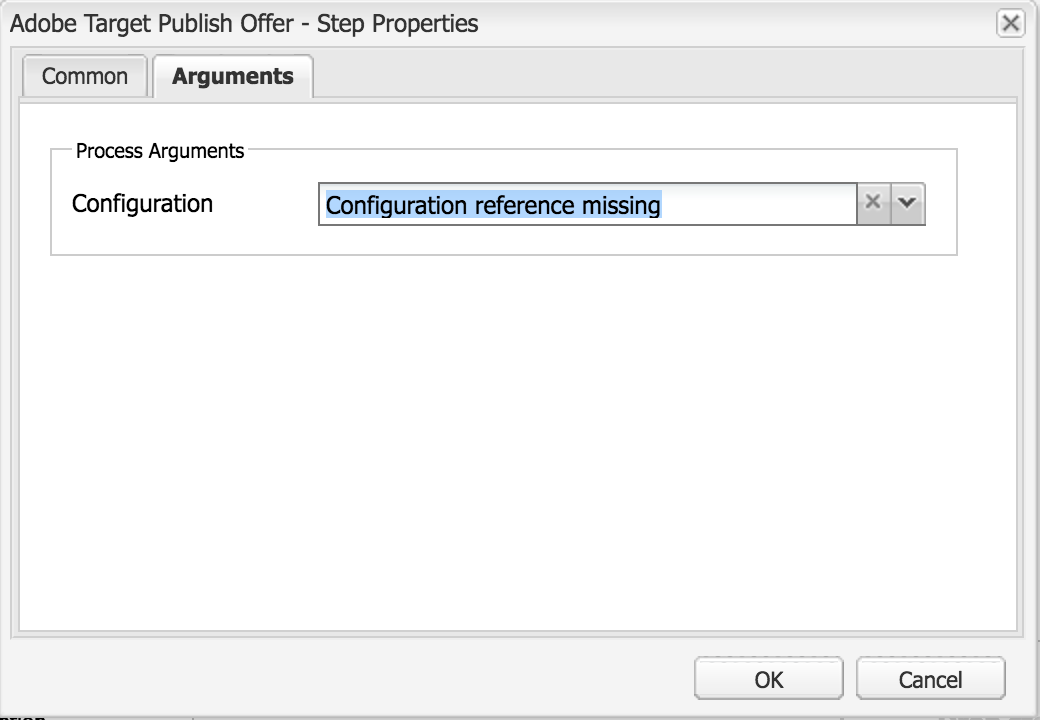
Test & Target
Instantiates a call to adobe's test and target system based on a test and target configuration
Unlock Payload Process
This is the opposite of the lock payload process
Unlock Payload Process
This is the opposite of the lock payload process
Workflow Initiator Participant Chooser
Chooses the initiator of the workflow as the next participant required to interact with the workflow
Chooses the initiator of the workflow as the next participant required to interact with the workflow
Workflow Offloading
From what I can tell this is a copy of the Generic Job Process
From what I can tell this is a copy of the Generic Job Process
Launchers
While you can write a workflow now, how does the workflow run? You can instantiate it manually in the console, or via code, however, AEM also gives a way to have a workflow run automatically. The process called launchers
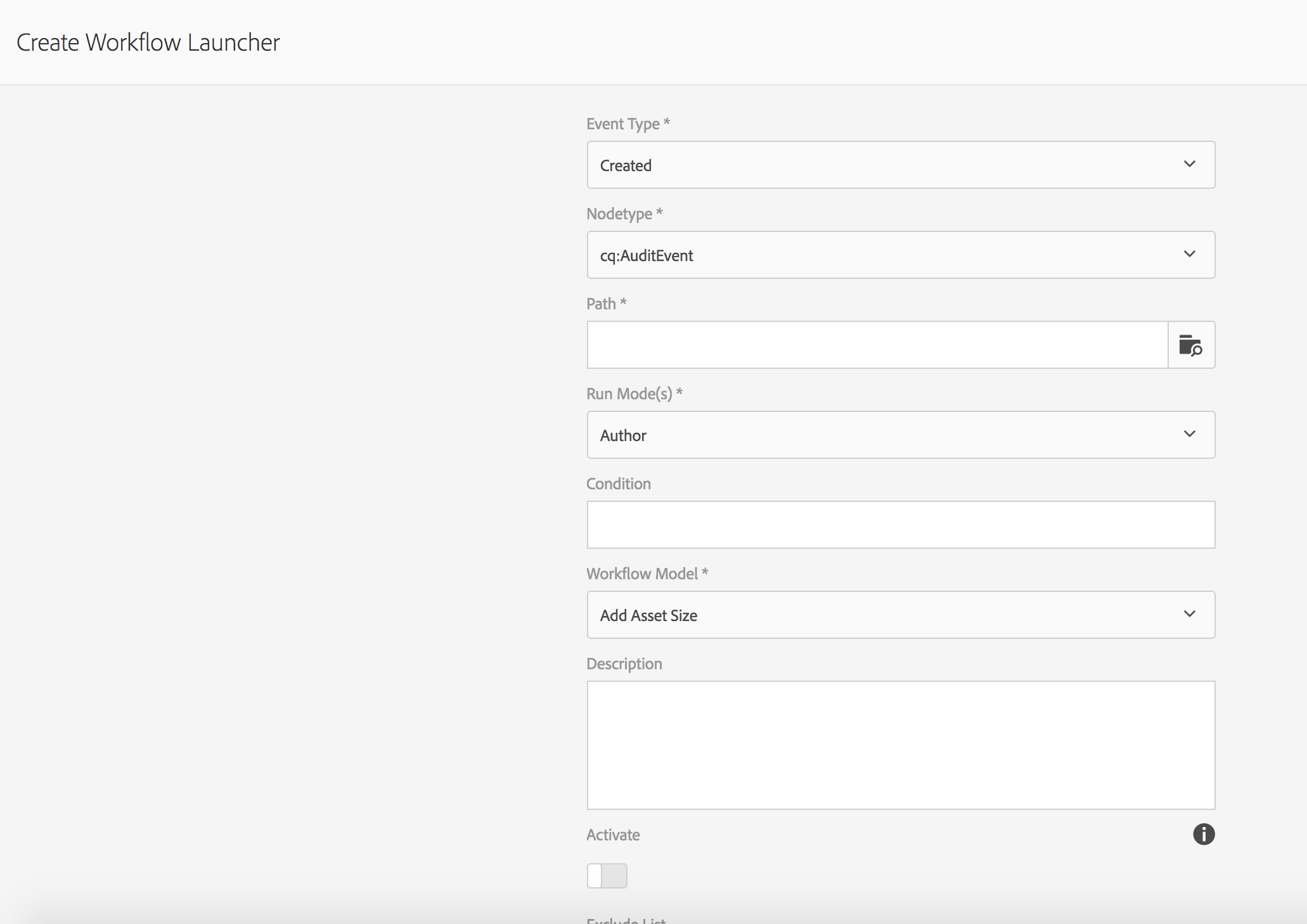
Workflow Launchers
Creating a launcher will allow you to specify when the workflow is run
While you can write a workflow now, how does the workflow run? You can instantiate it manually in the console, or via code, however, AEM also gives a way to have a workflow run automatically. The process called launchers
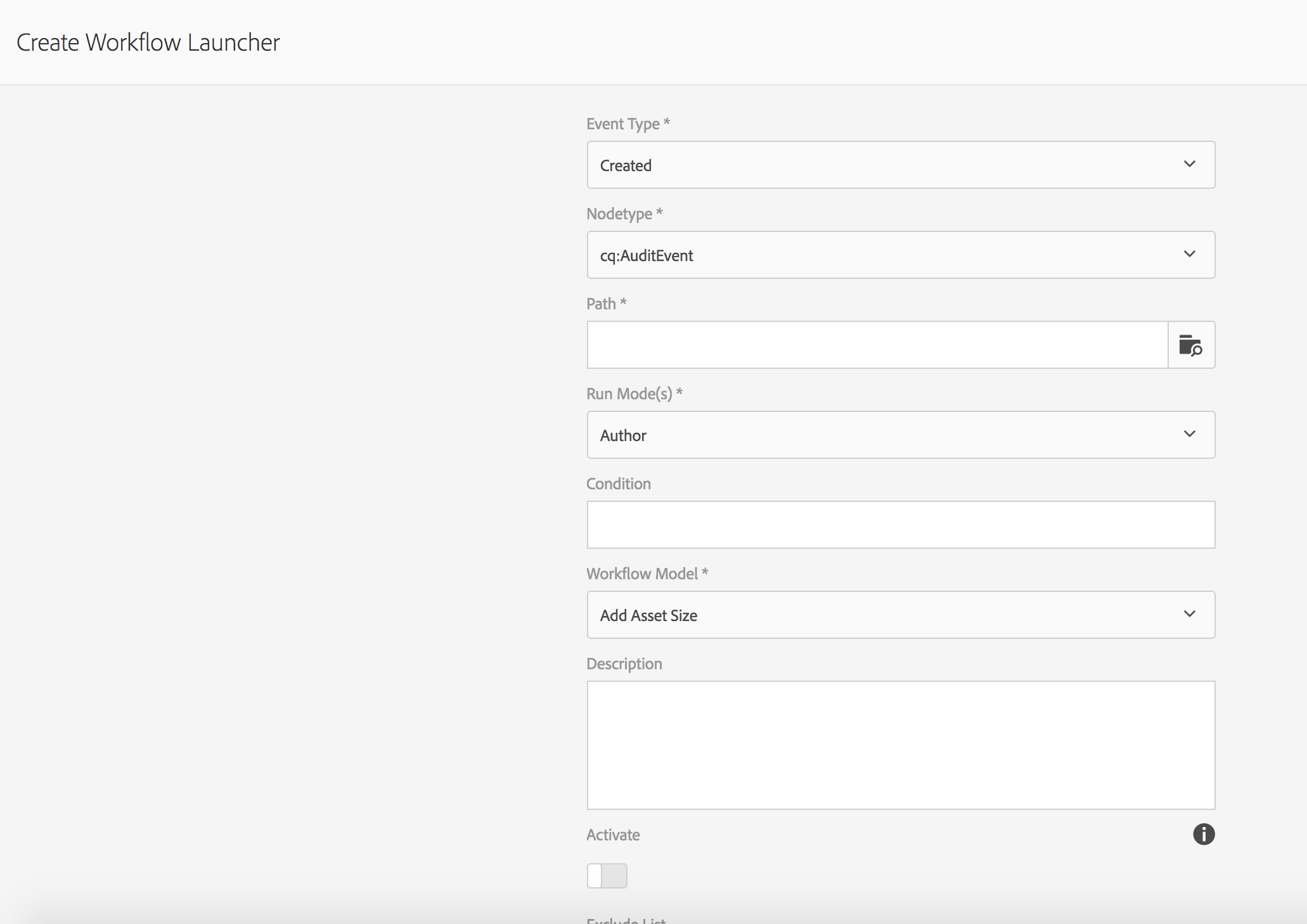
Workflow Launchers
Creating a launcher will allow you to specify when the workflow is run
- Event Type
- Determines what node changes cause the workflow to run
- Created
- Modified
- Removed
- Node Type
- What type of node are you interested in.
- There is a large selection of node types available in AEM to run a workflow against.
- If you are using custom nodes they will be nt:unstructured
- Path
- The path that the node needs to be within to launch the workflow
- Run Mode(s)
- Whether the workflow will run on the author, the publisher or both
- Condition
- An optional condition
- Can only be an equals match
- Tests properties on the node i.e. cq:template==/apps/geometrixx/templates/contentpage
- Workflow Model
- The model to run when the conditions match
- Description
- The description of what the launcher is doing
- Activate
- If the launcher is active
- Exclude List
- This specifies any JCR events to exclude (i.e. ignore) when determining whether a workflow should be triggered.
- This launcher property is a comma-separated list of items:
- property-name
- ignore any jcr event which triggered on the specified property name.
- event-user-data:<someValue>
- ignores any event that contains the <someValue> user-data set through the ObservationManager API.
For example:
- jcr:lastModified,dc:modified,dc:format,jcr:lastModifiedBy,imageMap,event-user-data:changedByWorkflowProcess
- This feature can be used to ignore any changes triggered by another workflow process by adding the exclude item:
- event-user-data:changedByWorkflowProcess
No comments:
Post a Comment
If you have any doubts or questions, please let us know.