Here are my 7 simple tips to make your JavaScript easier to read.
1. Avoid indirection.
- Your functions should return results instead of mutating their parameters.
- Prefer promises over callbacks.
Your code should flow in a single direction, consistently and predictably.
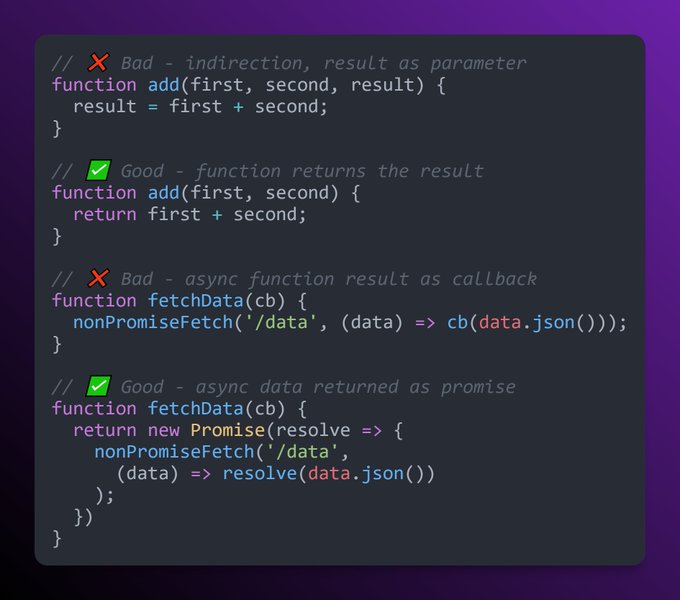
2. Use guard clauses to reduce nesting in conditions.
2. Use guard clauses to reduce nesting in conditions.
When you return inside of the if-statement, everything after that statement is basically an `else` clause. Take advantage of it to reduce excessive nesting.
3. Prevent ambiguous states.
When you model your state as a state machine, it becomes unambiguous, thus easier to read.
For example, if you have states such as isLoading, isLoaded, isSaving, and isError, they can't all be true at once. Use a string literal for that.
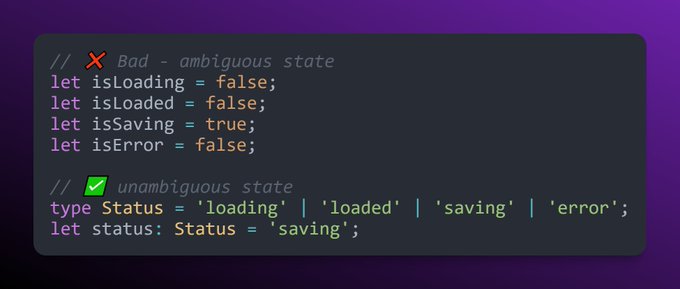
4. Handle promise errors.
4. Handle promise errors.
We tend to forget that things can fail for random reasons. Wrap your async calls with try/catch blocks and handle their failures properly.
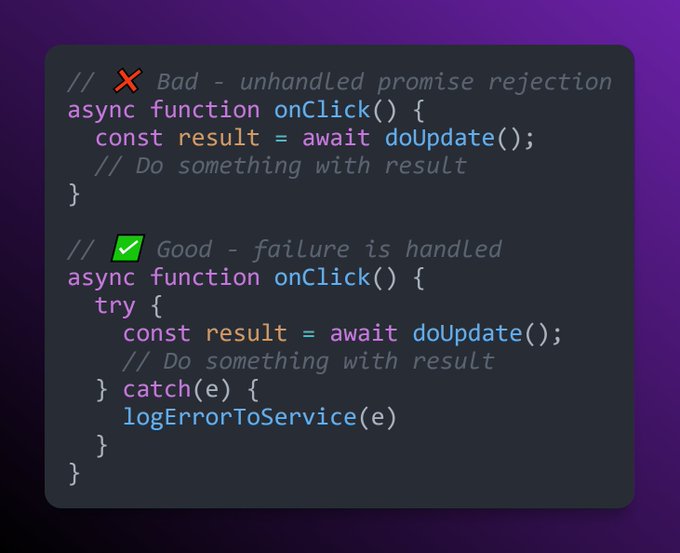
5. Replace switch/if-statements with object literal lookup.
When you use an if-statement to determine the value to use instead of directing control flow, the object literal lookup may be a better choice.
6. Prefer declarative APIs over "speed optimizations".
JavaScript arrays have declarative functions like `map()`, `filter()` or `find()`. You should use them instead of writing imperative, hard-to-read code, even when you can cram it all into a single for-loop.
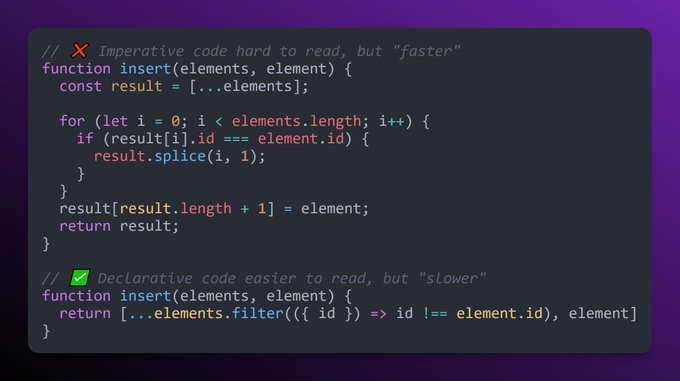
7. Name your function arguments.
When your function asks for more than 1-2 arguments, it becomes hard to follow which is which. Prefer a "config" object to provide names for function parameters.
Tip: when you use an object, some parameters can also be optional.
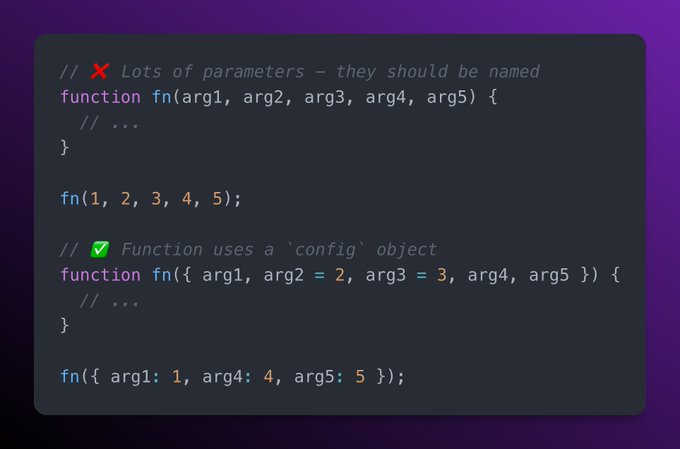
Source:
Vincas Stonys
@VincasStonys
Source:
Vincas Stonys
@VincasStonys
No comments:
Post a Comment
If you have any doubts or questions, please let us know.