How does JavaScript sort() method work?
Here is a small detailed ↓
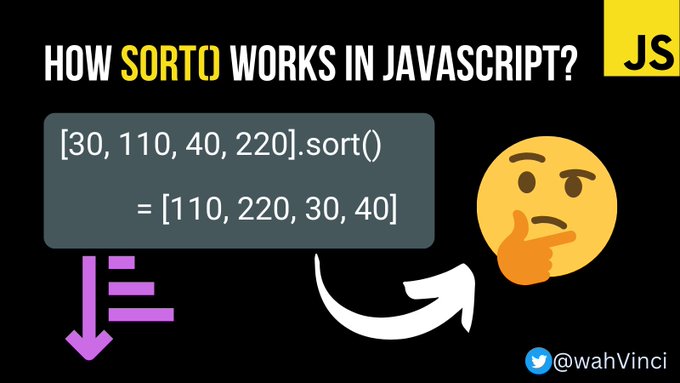
In JavaScript sorting is by default lexicographic & not numeric, regardless of the types of values in the array.
In JavaScript sorting is by default lexicographic & not numeric, regardless of the types of values in the array.
All values of the array will be treated as strings before sorting.
sort() method in JS actually modifies(mutates) the original array.
sort() method in JS actually modifies(mutates) the original array.
For example:
const nums = [30, 110, 40, 220];
nums.sort();
// nums: [110, 220, 30, 40]
For sorting arrays based on numeric values, it's always recommended to use a callback function.
For sorting arrays based on numeric values, it's always recommended to use a callback function.
Mutating the original array is not a good practice.
Learn more about mutating here↓
To avoid mutating the original array, duplicate the array using the slice() or spread operator and then sort the array with a callback function.
To avoid mutating the original array, duplicate the array using the slice() or spread operator and then sort the array with a callback function.
So a good way to sort the 'nums' array in the previous example would be ↓
const sortedArr = nums.slice().sort((a, b) => a - b);
const sortedArr = nums.slice().sort((a, b) => a - b);
// sortedArr: [30, 40, 110, 220]
// nums: [110, 220, 30, 40]
So sortedArr will be a new array that is sorted in ascending order without modifying the actual nums array.
And that is about sorting and best practice for sorting arrays in JavaScript.
And that is about sorting and best practice for sorting arrays in JavaScript.
No comments:
Post a Comment
If you have any doubts or questions, please let us know.